환율 가져오는것을 개발해본다. 환율 api가 횟수제한이 있기 때문에, 이미 가져왔다면 더 가져오지 않도록 한다.
그리고 환율 api가 토요일과 일요일에는 비어있기 때문에, 금요일 api를 가져오도록 한다.
export function getPreviousFriday(date:Date) {
const dayOfWeek = date.getDay(); // 0 (일요일)부터 6 (토요일)까지의 요일을 반환
const difference = dayOfWeek === 0 ? 2 : 1; // 일요일은 이틀 전, 토요일은 하루 전이 금요일
const previousFriday = new Date(date);
// 토요일 또는 일요일이면 지난 금요일로 이동
if (dayOfWeek === 0 || dayOfWeek === 6) {
previousFriday.setDate(date.getDate() - difference);
}
const year = previousFriday.getFullYear();
const month = String(previousFriday.getMonth() + 1).padStart(2, '0'); // 월은 0부터 시작하므로 +1 필요
const day = String(previousFriday.getDate()).padStart(2, '0');
return `${year}${month}${day}`;
}
한번 등록하면 2년동안 쓸 수 있다. 하루에 1000회 요청할 수 있다. 그래서 1000회 이상 요청하지 않도록 db를 활용해서 미리 db에 저장해두고, 이미 요청해서 db에 환율을 가져왔다면 추가로 요청하지 않도록 하였다.
https://www.koreaexim.go.kr/ir/HPHKIR020M01?apino=2&viewtype=C&searchselect=&searchword=
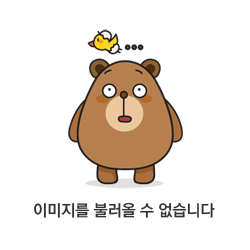
async function fetchExchangeRate(apiKey:string, searchDate: string) {
const url = `https://www.koreaexim.go.kr/site/program/financial/exchangeJSON?authkey=${apiKey}&searchdate=${searchDate}&data=AP01`;
try {
logger.info(`call api, apikey: ${apiKey}, date: ${searchDate}`);
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching exchange rate:', error);
}
}
'개발로 부자되기' 카테고리의 다른 글
firebase function 설치부터 배포까지 2023 (0) | 2023.09.01 |
---|---|
차트 레이싱 만들기 (0) | 2023.03.19 |
coingecko api 코인 가격 가져오기 (0) | 2023.03.19 |
nodejs XLSX 라이브러리, 엑셀 라이브러리 사용방법 (0) | 2023.03.10 |
구글 애드센스 next.js 웹페이지에 추가하기 (0) | 2023.02.17 |